ui Package¶
The UI sub-package contains graphical user interfaces for exploring the
toolbox functionality. The sub-package contains the Launcher
GUI which provides a list of
components and a brief description of their function. The rest of the
sub-package is split between simple
, utils
, tools
, iter
,
and examples
sub-packages providing interfaces to the OTSLM core
packages and examples of how the UI can be combined. The UI sub-package
also contains a otslm.ui.support
sub-package with
common code used by the GUIs.
This section provides information required to extend the Launcher, or other GUI windows as well as a brief overview of the other GUI components. For details on the functions the GUIs represent, see the corresponding package documentation: iter Package, tools Package, simple Package or utils Package. For details on how to use the GUIs, see Getting Started and Examples.
Launcher¶
-
otslm.ui.
Launcher
¶
The launcher consists of two layers: the category list and the
application list. The application list is populated when the user
selects a category. Details about the programs are specified in the
CategoryListBoxValueChanged
function and *Data
functions.
Specifying application names¶
Application names are specified in the CategoryListBoxValueChanged
function. To add a new application, extend the ItemsData
and
Items
fields of the ApplicationListBox
for the category you wish
to place the app in. The ItemsData
field is used in the *Data
function (see below) to get the application name and description.
Program name, description and launch command¶
Information about each of the programs is defined in the *Data
functions, one function for each sub-package: ExampleData
,
IterativeData
, ToolsData
, UtilitiesData
and SimpleData
.
These functions return a struct with the fields Name
,
Description
and AppName
for the user-readable name, description
and the Matlab application name to launch. The value returned depends on
the current value for the ApplicationListBox
list box. In order to
extend the applications list, simply add a new case to the switch for
the new application and set the corresponding values in the data
struct, for example:
data.Name = 'Mixing Two Beams';
data.Description = ['This example shows how to generate a phase only diffraction ' ...
'grating to split a beam into two independently controllable spots.'];
data.AppName = 'otslm.ui.examples.MixingTwoBeams';
Simple GUI overview¶
Most GUIs are split into 4 main sections, shown in Fig. 62
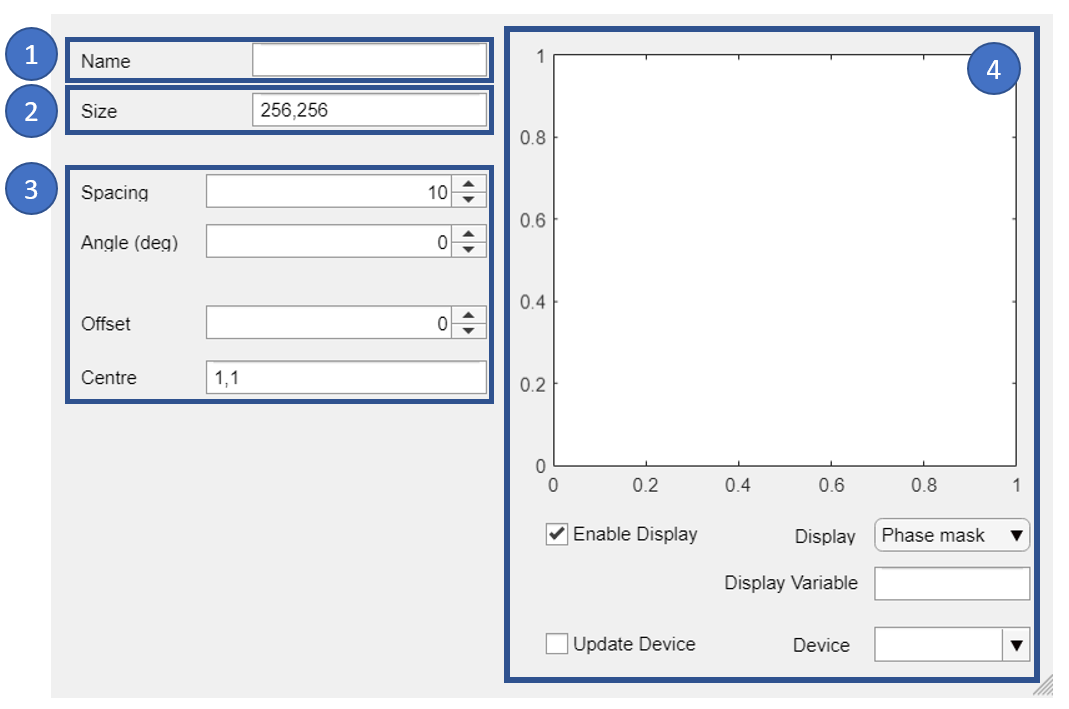
Fig. 62 Overview of ui.simple.linear graphical user interface.
The layout consists of:
(1) Output variable name;
(2) Size of pattern (mostly used on ui.simple.*
GUIs);
(3) Controls for the method; and
(4) Pattern preview window.
When the window launches it will search the base workspace for variables
names and otslm.utils.Showable
devices which can be used for
displaying the pattern (see otslm.ui.support.populateDeviceList()
).
Methods which updated as soon as the user changes a value will have most
of the implementation contained in a callback function. For
ui.simple.linear
, this is done in
otslm.ui.support.patternValueChanged()
.
The content of this function involves first getting the inputs
from the user and converting the strings to variables:
% Get the UI fileds for generating the pattern
name = app.NameEditField.Value;
sz = evalin('base', ['[', app.SizeEditField.Value, ']']);
spacing = app.SpacingSpinner.Value;
angle_deg = app.AngledegSpinner.Value;
offset = app.OffsetSpinner.Value;
centre = evalin('base', ['[', app.CentreEditField.Value, ']']);
The function then calls the OTSLM method:
% Generate the pattern
pattern = otslm.simple.linear(sz, spacing, ...
'centre', centre, 'angle_deg', angle_deg);
pattern = pattern + offset;
And finally, calls the
otslm.ui.support.simplePatternValueChanged()
helper
handle updating the preview window, saving the result to
the workspace and updating the device.
% Offload to the base class (sort of...)
otslm.ui.support.simplePatternValueChanged(name, pattern, ...
app.DeviceDropDown.Value, app.UpdateDeviceCheckBox.Value, ...
app.EnableDisplayCheckBox.Value, app.UIAxes, ...
app.DisplayDropDown.Value, app.DisplayVariableEditField.Value);
Most functions will have a public updateView
function which can be
used by other GUI windows to force an update to window after values have
changed.
Support sub-package¶
The support sub-package contains common code and functions used by the GUI components. These support functions can be used to design additional user interfaces using the toolbox. This section briefly describes these functions and how they are used by the existing GUI components.
Warning
Some of these functions should really be part of a custom GUI component layout class. To the best of our knowledge, this is currently not supported for Matlab Apps in R2018a. If this changes in a future Matlab release, much of this code will likely move/change.
Contents
calculateImageSliceFreq¶
-
otslm.ui.support.
calculateImageSliceFreq
(img, theta, offset, swidth)¶ Calculate the frequency spectrum of an image slice
- Usage
- [fvals, freqs] = calculateImageSliceFreq(img, theta, offset, swidth) calculates the frequency spectrum of a slice through an image.
- Parameters
- img – Real valued image to calculate spectrum from
- theta – Angle of slice (radians)
- offset – Offset of slice (pixels)
- swidth – width of slice (pixels) to average over
- Returns
- fvals – Calculated amplitudes
- freqs – Corresponding frequencies
This function is used for the power spectrum plots in the calibration functions. The function samples a slice of pixels from an image. Arguments control the slice position, width and angle. The function returns the spatial frequencies and complex amplitudes. For example usage, see
ui.utils.CalibrationStepFarfield
.
checkImagesChanged¶
-
otslm.ui.support.
checkImagesChanged
(oldImages, newImages)¶ Compare two cell arrays of images for changes
- Usage
- changed = checkImagesChanged(oldImage, newImages) compares each image in the two cell arrays for differences. If the cell arrays are different, returns true.
- Parameters
- oldImages – first cell array of images to compare
- newImages – second cell array of images to compare
- Returns
- changed (logical) – if the images have changed
This function is used by most methods which have an input image, including
ui.tools.Visualise
,ui.tools.Finalize
andui.tools.Dither
. The two inputs contain cell arrays of matrices to be compared. If either the length of the cell arrays, size or type of the images, or the image data are different, the function returns true. This can be a expensive comparison. We look for changes between the old and new images rather than watching for a change event on variables, this is to allow the user to enter constants or procedural functions into the GUI inputs.
cleanTimer¶
-
otslm.ui.support.
cleanTimer
(tmr)¶ Cleans up the timer when the app is about to finish
- Usage
- cleanTimer(tmr) attempts to delete the specified timer.
- Parameters
- tmr – The matlab timer to clean up
This function shouldn’t intentionally raise any warnings.
Function attempts to stop and delete the given timer. The function avoids raising errors, making it safe to use in a GUI clean-up method. Timers are mainly used to watch for changes to input variables, such as image inputs to
ui.tools.Visualise
,ui.tools.Finalize
andui.tools.Dither
.
complexPatternValueChanged¶
-
otslm.ui.support.
complexPatternValueChanged
(name, phase, amplitude, ptype, device_name, enable_update, enable_display, display_ax, display_type, display_name)¶ Common code for simple update uis with ptype
- Usage
- complexPatternValueChanged(name, phase, amplitude, ptype, device_name, enable_update, enable_display, display_ax, display_type, display_name)
- Parameters
- name – Variable name to save pattern in base workspace.
- phase – Phase part of pattern.
- amplitude – Amplitude part of pattern
- ptype – type of pattern. Must be ‘phase’, ‘amplitude’ or ‘complex’.
- device_name – Name of Showable device to display pattern on
- enable_update – If showable device should be updated
- enable_display – If preview should be displayed
- display_ax – Axis for preview
- display_type – Type argument for display,
see
updateComplexDisplay()
for options. - display_name – Output variable name for preview data
This should realy be part of the base class, but we don’t seem to be able to package apps with a custom base class. Maybe in future MATLAB versions this might be possible.
As per
simplePatternValueChanged()
but with complex patterns and an additionalptype
argument.See also
iterPatternValueChanged()
andupdateComplexDisplay()
.
findTabUserdata¶
-
otslm.ui.support.
findTabUserdata
(tab, tag)¶ Find entries with the specific user-data tag and returns a struct
- Usage
- userdata = findTabUserdata(tab, tag)
- Parameters
- tab – An object which can be passed to
findall
- tag – Cell array of values for UserData property to search for
- tab – An object which can be passed to
- Returns
struct
with fields corresponding totag
values
This function uses
findall
to search the givenTab
for entries whoseUserData
attribute is set to one of the specified strings.tag
should be a cell array of character vectors for the tags to search for. Example usage (based onui.tools.SampleRegion
):
getDeviceFromBase¶
-
otslm.ui.support.
getDeviceFromBase
(sname)¶ Get an showable object from the base workspace
- Usage
- slm = getDeviceFromBase(sname)
- Parameters
- sname – string for device variable name in base workspace
- Returns
- Returns the Showable device or an empty list.
This function attempts to get the variable specified by
sname
from the base workspace. Ifsname
is empty, the function returns an empty matrix. Ifsname
is not a variable name, the function raises a warning. Otherwise, the function gets the variable and checks to see if it is valid usingisvalid
. For example usage seesimplePatternValueChanged()
.
getImageOrNone¶
-
otslm.ui.support.
getImageOrNone
(name, silent)¶ Get the image from the base workspace or an empty array
- Usage
im = getImageOrNone(name) gets the variable name from base or None if any error occurs.
im = getImageOrNone(name, silent) as above but if
silent=true
does not retrow the error to the console, just silently ignores it.- Parameters
- name – variable name for image in base workspace
- silent (logical) – True if the method should not print warnings
Attempts to evaluate the given string in the base workspace with
evalin
. The string can either be a variable name or valid matlab code which can be evaluated in the users base workspace.If an error occurs, the function prints the error to the console and returns a empty matrix. If the silent argument is set to true, the function does not print to the console (useful for methods which frequently check for the existence of a variable, such as
checkImagesChanged()
. For example usage, seeui.tools.Visualise
,ui.tools.finalize
andui.tools.dither
.
iterPatternValueChanged¶
-
otslm.ui.support.
iterPatternValueChanged
(name, pattern, device_name, enable_update, enable_display, display_ax, display_type, display_name, fitness_method)¶ Common code for iter update uis
- Usage
- iterPatternValueChanged(name, pattern, … device_name, enable_update, enable_display, … display_ax, display_type, display_name, fitness_method)
- Parameters
- name – Variable name to save pattern in base workspace.
- pattern – Pattern to display/preview
- device_name – Name of Showable device to display pattern on
- enable_update – If showable device should be updated
- enable_display – If preview should be displayed
- display_ax – Axis for preview
- display_type – Type argument for display,
see
updateIterDisplay()
for options. - display_name – Output variable name for preview data
- fitness_method – Function handle for fitness graph
This should realy be part of the base class, but we don’t seem to be able to package apps with a custom base class. Maybe in future MATLAB versions this might be possible.
Function is similar to
simplePatternValueChanged()
but with a function handle for plotting fitness scores.See also See also
complexPatternValueChanged()
andupdateIterDisplay()
.
populateDeviceList¶
-
otslm.ui.support.
populateDeviceList
(list, type_name)¶ Populates the device list with Showable devices
- Usage
populateDeviceList(list) populates the device drop down list with the
otslm.utils.Showable
devices in the base workspace.populateDeviceList(list, type_name) specify types of devices to populate list with.
- Parameters
- list (uidropdown) – List handle to add items to
- type_name – Name of type to filter variables
by (optional, default:
otslm.utils.Showable
)
This function is used to populate the contents of a
uidropdown
widget. The function takes a handle to theuidropdown
widget, an optional Matlab class name and searches the base workspace for variables with the specified type. If no class name is specified, the method populates the list withShowable
object names. For example usage, seeui.simple.linear
.
saveVariableToBase¶
-
otslm.ui.support.
saveVariableToBase
(name, pattern, warn_prefix)¶ Saves the variables to the base workspace
- Usage
- saveVariableToBase(name, pattern, warn_prefix) Saves the variable pattern to the base workspace with variable name name. If name is invalid, prefixes warning with warn_prefix.
- Parameters
- name – variable name to save pattern to
- pattern – pattern to be saved
- warn_prefix – prefix to add to warnings
If the name is empty, aborts the operation. If the names is an invalid variable name, raises a warning.
This function is used by most GUIs for saving computed patterns into the base workspace, for example usage see
simplePatternValueChanged()
.
simplePatternValueChanged¶
-
otslm.ui.support.
simplePatternValueChanged
(name, pattern, device_name, enable_update, enable_display, display_ax, display_type, display_name)¶ Common code for simple update uis
- Usage
- simplePatternValueChanged(name, pattern, … device_name, enable_update, enable_display, … display_ax, display_type, display_name)
- Parameters
- name – Variable name to save pattern in base workspace.
- pattern – Pattern to save/preview/display
- device_name – Name of Showable device to display pattern on
- enable_update – If showable device should be updated
- enable_display – If preview should be displayed
- display_ax – Axis for preview
- display_type – Type argument for display,
see
updateSimpleDisplay()
for options. - display_name – Output variable name for preview data
This should realy be part of the base class, but we don’t seem to be able to package apps with a custom base class. Maybe in future MATLAB versions this might be possible.
This function is used by most of the simple GUIs including
ui.simple.linear
,ui.simple.random
, andui.tools.combine
. The function takes as input values from the various GUI components as well as the generated pattern. The function saves the pattern to the workspace, displays the pattern on the device, and updates the pattern preview (if the appropriate values are set).See also
iterPatternValueChanged()
andcomplexPatternValueChanged()
.
updateComplexDisplay¶
-
otslm.ui.support.
updateComplexDisplay
(pattern, slm, ptype, display_type, ax, output_name)¶ Helper for the display on simple uis with ptype
- Usage
- updateComplexDisplay(pattern, slm, ptype, display_type, ax, output_name) Generates the display pattern, updates the axis and outputs to base.
- Parameters
- pattern – pattern to be displayed
- slm – showable device displaying pattern (or
[]
) - ptype – type of pattern. Must be ‘phase’, ‘amplitude’ or ‘complex’.
- display_type – mode for the preview window. can be ‘phase’, ‘raw’, ‘device’, or ‘farfield’.
- ax – axis to place the preview in
- output_name – output variable name in base workspace (or
[]
)
As per
updateSimpleDisplay()
but with complex patterns and an additionalptype
argument.See also
updateIterDisplay()
andcomplexPatternValueChanged()
updateIterDisplay¶
-
otslm.ui.support.
updateIterDisplay
(pattern, slm, display_type, ax, output_name, fitness_method)¶ Helper for updating the display on iterative uis.
- Usage
- updateIterDisplay(pattern, slm, display_type, ax, output_name, fitness_method) generates the display pattern, updates the axis and outputs to base.
- Parameters
- pattern – pattern to be displayed
- slm – showable device displaying pattern (or
[]
) - display_type – mode for the preview window. can be ‘phase’, ‘error’, ‘device’, or ‘farfield’
- ax – axis to place the preview in
- output_name – output variable name in base workspace (or
[]
) - fitness_method – function to plot fitness
Similar to
updateSimpleDisplay()
but displays either the phase pattern, error function, simulated far-field or device pattern in the preview window.This function generates the pattern to display in the preview axis. If
output_name
is not empty, the function also writes the pattern to the specified variable name.See also
updateComplexDisplay()
anditerPatternValueChanged()
.
updateSimpleDisplay¶
-
otslm.ui.support.
updateSimpleDisplay
(pattern, slm, display_type, ax, output_name)¶ Helper for updating the display on simple uis.
- Usage
- updateSimpleDisplay(pattern, slm, display_type, ax, output_name) Generates the display pattern, updates the axis and outputs to the base workspace.
- Parameters
- pattern – pattern to be displayed
- slm – showable device displaying pattern (or
[]
) - display_type – mode for the preview window. can be ‘Phase mask’, ‘Raw phase mask’, ‘Device image’, or ‘Simulated farfield’
- ax – axis to place the preview in
- output_name – output variable name in base workspace (or
[]
)
This function generates the pattern to display in the preview axis. If
output_name
is not empty, the function also writes the pattern to the specified variable name.This function is used by most of the simple GUIs including
ui.simple.linear
,ui.simple.random
, andui.tools.combine
. For example usage, seesimplePatternValueChanged()
.See also
updateComplexDisplay()
andupdateIterDisplay()
.